Identifying Special Characters in a Given String
-------------------------------------------------------------------------------------------------
package special;
public class IdentifySpecialChar {
/**
* @param args
*/
public static void main(String[] args) {
try {
System.out.println(IdentifySpecialChar.identifyingSpecialCharacters("Gangadhar"));
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/**
* This method is used to identify the special characters in the given value,
* if there are any special characters it return true else return false.
* @param value
* @return
* @throws Exception
*/
public static boolean identifyingSpecialCharacters(String value) throws Exception {
boolean returnVal = false;
char[] charArray = value.toCharArray();
for(int i=0;i< charArray.length;i++) {
System.out.println((int)charArray[i]);
if( (((int)charArray[i]) >= 32 && ((int)charArray[i]) <= 47) ||
(((int)charArray[i]) >= 58 && ((int)charArray[i]) <= 64) ||
(((int)charArray[i]) >= 91 && ((int)charArray[i]) <= 96) ||
(((int)charArray[i]) >= 123 && ((int)charArray[i]) <= 127)) {
returnVal = true;
break;
}
}
return returnVal;
}
}
Monday, March 29, 2010
Thursday, February 18, 2010
Hibernate - OneToOne
***************************************************************************************
Tables
***************************************************************************************
create table "SCOTT"."PERSON"(
"PERSON_ID" NUMBER(10) not null,
"PERSON_NAME" VARCHAR2(20),
constraint "PK_PERSON" primary key ("PERSON_ID")
);
create unique index "PK_PERSON" on "SCOTT"."PERSON"("PERSON_ID");
create table "SCOTT"."COMPUTER"(
"COM_ID" NUMBER(10) not null,
"COMPUTER_NAME" VARCHAR2(20),
"PROCESSOR" VARCHAR2(20),
"PERSON_ID" NUMBER(10) unique,
constraint "PK_COMPUTER" primary key ("COM_ID")
);
alter table "SCOTT"."COMPUTER"
add constraint "FK_PERSON_ID"
foreign key ("PERSON_ID")
references "SCOTT"."PERSON"("PERSON_ID");
create unique index "PK_COMPUTER" on "SCOTT"."COMPUTER"("COM_ID");
create unique index "UNIQUE_PERID" on "SCOTT"."COMPUTER"("PERSON_ID");
***************************************************************************************
Hibernate Factory Class
------------------------
package factory;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal threadLocal = new ThreadLocal();
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the
*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
***************************************************************************************
Pojo Classes
------------
package model;
// default package
/**
* Computer generated by MyEclipse - Hibernate Tools
*/
public class Computer implements java.io.Serializable {
// Fields
private Long comId;
private Person person;
private String computerName;
private String processor;
// Constructors
/** default constructor */
public Computer() {
}
/** full constructor */
public Computer(Person person, String computerName, String processor) {
this.person = person;
this.computerName = computerName;
this.processor = processor;
}
// Property accessors
public Long getComId() {
return this.comId;
}
public void setComId(Long comId) {
this.comId = comId;
}
public Person getPerson() {
return this.person;
}
public void setPerson(Person person) {
this.person = person;
}
public String getComputerName() {
return this.computerName;
}
public void setComputerName(String computerName) {
this.computerName = computerName;
}
public String getProcessor() {
return this.processor;
}
public void setProcessor(String processor) {
this.processor = processor;
}
}
package model;
// default package
import java.util.HashSet;
import java.util.Set;
/**
* Person generated by MyEclipse - Hibernate Tools
*/
public class Person implements java.io.Serializable {
// Fields
private Long personId;
private String personName;
private Set computers = new HashSet(0);
// Constructors
/** default constructor */
public Person() {
}
/** full constructor */
public Person(String personName, Set computers) {
this.personName = personName;
this.computers = computers;
}
// Property accessors
public Long getPersonId() {
return this.personId;
}
public void setPersonId(Long personId) {
this.personId = personId;
}
public String getPersonName() {
return this.personName;
}
public void setPersonName(String personName) {
this.personName = personName;
}
public Set getComputers() {
return this.computers;
}
public void setComputers(Set computers) {
this.computers = computers;
}
}
***************************************************************************************
Mapping Files
-------------- 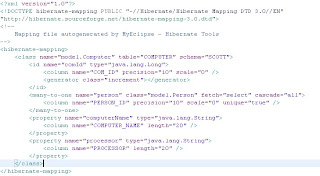
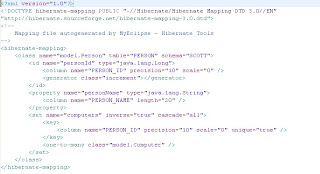
***************************************************************************************
DAO Class
-----------
package dao;
import java.io.Serializable;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Session;
import org.hibernate.Transaction;
import factory.HibernateSessionFactory;
public class OneToOneDAO {
public Object saveComputer(Class clazz, Object object) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Serializable id = session.save(object);
tx.commit();
Object returnObj = session.get(clazz, id, LockMode.UPGRADE);
return returnObj;
}
}
***************************************************************************************
Test Class
----------
package test;
import java.util.HashSet;
import java.util.Set;
import dao.OneToOneDAO;
import model.Computer;
import model.Person;
public class TestOneToOne {
/**
* @param args
*/
public static void main(String[] args) {
OneToOneDAO oneToOneDAO = new OneToOneDAO();
Computer computer = new Computer();
computer.setComputerName("Gr_Computer");
computer.setProcessor("Intel Duo Core");
Person person = new Person();
person.setPersonName("Gangadhar");
/*Person person1 = new Person();
person1.setPersonName("Rao");*/
computer.setPerson(person);
// Here in Computer class you can able to set only one person object, you can not set more than one.
/*computer.setPerson(person1);*/
Set setOfComputers = new HashSet();
setOfComputers.add(computer);
person.setComputers(setOfComputers);
/*person1.setComputers(setOfComputers);*/
oneToOneDAO.saveComputer(Computer.class, computer);
}
}
***************************************************************************************
Tables
***************************************************************************************
create table "SCOTT"."PERSON"(
"PERSON_ID" NUMBER(10) not null,
"PERSON_NAME" VARCHAR2(20),
constraint "PK_PERSON" primary key ("PERSON_ID")
);
create unique index "PK_PERSON" on "SCOTT"."PERSON"("PERSON_ID");
create table "SCOTT"."COMPUTER"(
"COM_ID" NUMBER(10) not null,
"COMPUTER_NAME" VARCHAR2(20),
"PROCESSOR" VARCHAR2(20),
"PERSON_ID" NUMBER(10) unique,
constraint "PK_COMPUTER" primary key ("COM_ID")
);
alter table "SCOTT"."COMPUTER"
add constraint "FK_PERSON_ID"
foreign key ("PERSON_ID")
references "SCOTT"."PERSON"("PERSON_ID");
create unique index "PK_COMPUTER" on "SCOTT"."COMPUTER"("COM_ID");
create unique index "UNIQUE_PERID" on "SCOTT"."COMPUTER"("PERSON_ID");
***************************************************************************************
Hibernate Factory Class
------------------------
package factory;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the
SessionFactory
if needed.*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
***************************************************************************************
Pojo Classes
------------
package model;
// default package
/**
* Computer generated by MyEclipse - Hibernate Tools
*/
public class Computer implements java.io.Serializable {
// Fields
private Long comId;
private Person person;
private String computerName;
private String processor;
// Constructors
/** default constructor */
public Computer() {
}
/** full constructor */
public Computer(Person person, String computerName, String processor) {
this.person = person;
this.computerName = computerName;
this.processor = processor;
}
// Property accessors
public Long getComId() {
return this.comId;
}
public void setComId(Long comId) {
this.comId = comId;
}
public Person getPerson() {
return this.person;
}
public void setPerson(Person person) {
this.person = person;
}
public String getComputerName() {
return this.computerName;
}
public void setComputerName(String computerName) {
this.computerName = computerName;
}
public String getProcessor() {
return this.processor;
}
public void setProcessor(String processor) {
this.processor = processor;
}
}
package model;
// default package
import java.util.HashSet;
import java.util.Set;
/**
* Person generated by MyEclipse - Hibernate Tools
*/
public class Person implements java.io.Serializable {
// Fields
private Long personId;
private String personName;
private Set computers = new HashSet(0);
// Constructors
/** default constructor */
public Person() {
}
/** full constructor */
public Person(String personName, Set computers) {
this.personName = personName;
this.computers = computers;
}
// Property accessors
public Long getPersonId() {
return this.personId;
}
public void setPersonId(Long personId) {
this.personId = personId;
}
public String getPersonName() {
return this.personName;
}
public void setPersonName(String personName) {
this.personName = personName;
}
public Set getComputers() {
return this.computers;
}
public void setComputers(Set computers) {
this.computers = computers;
}
}
***************************************************************************************
Mapping Files
--------------
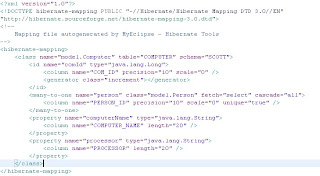
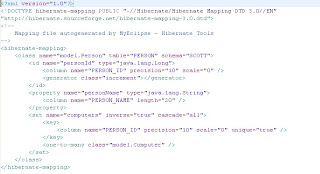
DAO Class
-----------
package dao;
import java.io.Serializable;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Session;
import org.hibernate.Transaction;
import factory.HibernateSessionFactory;
public class OneToOneDAO {
public Object saveComputer(Class clazz, Object object) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Serializable id = session.save(object);
tx.commit();
Object returnObj = session.get(clazz, id, LockMode.UPGRADE);
return returnObj;
}
}
***************************************************************************************
Test Class
----------
package test;
import java.util.HashSet;
import java.util.Set;
import dao.OneToOneDAO;
import model.Computer;
import model.Person;
public class TestOneToOne {
/**
* @param args
*/
public static void main(String[] args) {
OneToOneDAO oneToOneDAO = new OneToOneDAO();
Computer computer = new Computer();
computer.setComputerName("Gr_Computer");
computer.setProcessor("Intel Duo Core");
Person person = new Person();
person.setPersonName("Gangadhar");
/*Person person1 = new Person();
person1.setPersonName("Rao");*/
computer.setPerson(person);
// Here in Computer class you can able to set only one person object, you can not set more than one.
/*computer.setPerson(person1);*/
Set
setOfComputers.add(computer);
person.setComputers(setOfComputers);
/*person1.setComputers(setOfComputers);*/
oneToOneDAO.saveComputer(Computer.class, computer);
}
}
Wednesday, February 17, 2010
Hibernate - Many To One Example
****************************************************************************************
Tables
****************************************************************************************
create table "SCOTT"."ADD_RESS"(
"ADDRESS_ID" NUMBER(10) not null,
"ADDRESS_STREET" VARCHAR2(20),
"ADDRESS_CITY" VARCHAR2(20),
"ADDRESS_STATE" VARCHAR2(20),
"ADDRESS_PIN" NUMBER(10),
constraint "PK_ADD_RESS" primary key ("ADDRESS_ID")
);
create unique index "PK_ADD_RESS" on "SCOTT"."ADD_RESS"("ADDRESS_ID");
create table "SCOTT"."STUDENT"(
"STUDENT_ID" NUMBER(10) not null,
"STUDENT_NAME" VARCHAR2(30),
"STUDENT_ADDRESS" NUMBER(10),
constraint "PK_STUDENT" primary key ("STUDENT_ID")
);
alter table "SCOTT"."STUDENT"
add constraint "ADD_FK"
foreign key ("STUDENT_ADDRESS")
references "SCOTT"."ADD_RESS"("ADDRESS_ID");
create unique index "PK_STUDENT" on "SCOTT"."STUDENT"("STUDENT_ID");
****************************************************************************************
Hibernate Session Factory Class
****************************************************************************************
package factory;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal threadLocal = new ThreadLocal();
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the
*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
****************************************************************************************
HibernateCFG.xml File
****************************************************************************************
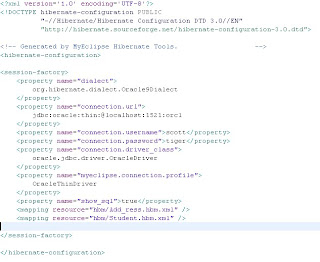
****************************************************************************************
Pojo Classes
****************************************************************************************
package model;
// default package
/**
* Student generated by MyEclipse - Hibernate Tools
*/
public class Student implements java.io.Serializable {
// Fields
private Long studentId;
private Add_ress addRess;
private String studentName;
// Constructors
/** default constructor */
public Student() {
}
/** full constructor */
public Student(Add_ress addRess, String studentName) {
this.addRess = addRess;
this.studentName = studentName;
}
// Property accessors
public Long getStudentId() {
return this.studentId;
}
public void setStudentId(Long studentId) {
this.studentId = studentId;
}
public Add_ress getAddRess() {
return this.addRess;
}
public void setAddRess(Add_ress addRess) {
this.addRess = addRess;
}
public String getStudentName() {
return this.studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
}
package model;
// default package
import java.util.HashSet;
import java.util.Set;
/**
* Add_ress generated by MyEclipse - Hibernate Tools
*/
public class Add_ress implements java.io.Serializable {
// Fields
private Long addressId;
private String addressStreet;
private String addressCity;
private String addressState;
private Long addressPin;
private Set students = new HashSet(0);
// Constructors
/** default constructor */
public Add_ress() {
}
/** full constructor */
public Add_ress(String addressStreet, String addressCity, String addressState, Long addressPin, Set students) {
this.addressStreet = addressStreet;
this.addressCity = addressCity;
this.addressState = addressState;
this.addressPin = addressPin;
this.students = students;
}
// Property accessors
public Long getAddressId() {
return this.addressId;
}
public void setAddressId(Long addressId) {
this.addressId = addressId;
}
public String getAddressStreet() {
return this.addressStreet;
}
public void setAddressStreet(String addressStreet) {
this.addressStreet = addressStreet;
}
public String getAddressCity() {
return this.addressCity;
}
public void setAddressCity(String addressCity) {
this.addressCity = addressCity;
}
public String getAddressState() {
return this.addressState;
}
public void setAddressState(String addressState) {
this.addressState = addressState;
}
public Long getAddressPin() {
return this.addressPin;
}
public void setAddressPin(Long addressPin) {
this.addressPin = addressPin;
}
public Set getStudents() {
return this.students;
}
public void setStudents(Set students) {
this.students = students;
}
}
****************************************************************************************
Mapping Files
****************************************************************************************
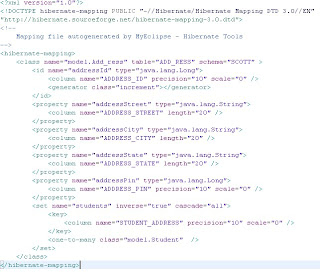
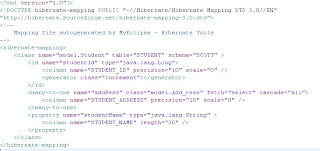
****************************************************************************************
Actual DAO Class
****************************************************************************************
package test;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Set;
import model.Add_ress;
import model.Student;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Session;
import org.hibernate.Transaction;
import factory.HibernateSessionFactory;
public class TestManyToOne {
/**
* @param args
*/
public static void main(String[] args) {
//Saving Address and Student Objects
Add_ress add_ress = new Add_ress();
add_ress.setAddressCity("Yanam");
add_ress.setAddressState("Pondicherry");
add_ress.setAddressStreet("1st Cross");
add_ress.setAddressPin(533464l);
Student student = new Student();
student.setStudentName("Swetha");
Student student1 = new Student();
student1.setStudentName("Nagendra");
student.setAddRess(add_ress);
student1.setAddRess(add_ress);
Set studentSet = new HashSet();
studentSet.add(student);
studentSet.add(student1);
add_ress.setStudents(studentSet);
// new TestManyToOne().saveStudent(Add_ress.class, add_ress);
//Saving Address and Student Objects
/********************************************************************/
//Getting Address and Student Objects.
/*Add_ress add_ress1 = new Add_ress();
add_ress1.setAddressId(4l);
add_ress1 = (Add_ress)new TestManyToOne().getAddress(Add_ress.class, add_ress1.getAddressId());
System.out.println("City : " + add_ress1.getAddressCity());
System.out.println("State : " + add_ress1.getAddressState());
System.out.println("Street: " + add_ress1.getAddressStreet());
System.out.println("PIN : " + add_ress1.getAddressPin());
studentSet = add_ress1.getStudents();
for (Student student2 : studentSet) {
System.out.println("--------------------------------------------");
System.out.println("Student ID : " + student2.getStudentId());
System.out.println("Student Name: " + student2.getStudentName());
}*/
//Getting Address and Student Objects.
/********************************************************************/
Add_ress add_ress2 = new Add_ress();
Set stdSet = new HashSet();
Student std1 = new Student();
std1.setStudentId(5l);
Student std2 = new Student();
std2.setStudentId(6l);
stdSet.add(std1);
stdSet.add(std2);
add_ress2.setAddressId(4l);
add_ress2.setStudents(stdSet);
new TestManyToOne().deleteAddress(add_ress2);
}
public void saveStudent(Class clazz, Object object) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Serializable id = session.save(object);
session.close();
tx.commit();
}
public Object getAddress(Class clazz, long id) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Object returnObj = session.get(clazz, id, LockMode.UPGRADE);
return returnObj;
}
public void deleteAddress(Add_ress add_ress) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
session.delete(add_ress);
//session.close();
tx.commit();
}
}
****************************************************************************************
Tables
****************************************************************************************
create table "SCOTT"."ADD_RESS"(
"ADDRESS_ID" NUMBER(10) not null,
"ADDRESS_STREET" VARCHAR2(20),
"ADDRESS_CITY" VARCHAR2(20),
"ADDRESS_STATE" VARCHAR2(20),
"ADDRESS_PIN" NUMBER(10),
constraint "PK_ADD_RESS" primary key ("ADDRESS_ID")
);
create unique index "PK_ADD_RESS" on "SCOTT"."ADD_RESS"("ADDRESS_ID");
create table "SCOTT"."STUDENT"(
"STUDENT_ID" NUMBER(10) not null,
"STUDENT_NAME" VARCHAR2(30),
"STUDENT_ADDRESS" NUMBER(10),
constraint "PK_STUDENT" primary key ("STUDENT_ID")
);
alter table "SCOTT"."STUDENT"
add constraint "ADD_FK"
foreign key ("STUDENT_ADDRESS")
references "SCOTT"."ADD_RESS"("ADDRESS_ID");
create unique index "PK_STUDENT" on "SCOTT"."STUDENT"("STUDENT_ID");
****************************************************************************************
Hibernate Session Factory Class
****************************************************************************************
package factory;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the
SessionFactory
if needed.*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
****************************************************************************************
HibernateCFG.xml File
****************************************************************************************
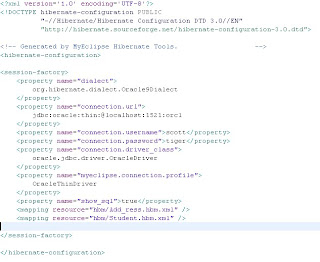
****************************************************************************************
Pojo Classes
****************************************************************************************
package model;
// default package
/**
* Student generated by MyEclipse - Hibernate Tools
*/
public class Student implements java.io.Serializable {
// Fields
private Long studentId;
private Add_ress addRess;
private String studentName;
// Constructors
/** default constructor */
public Student() {
}
/** full constructor */
public Student(Add_ress addRess, String studentName) {
this.addRess = addRess;
this.studentName = studentName;
}
// Property accessors
public Long getStudentId() {
return this.studentId;
}
public void setStudentId(Long studentId) {
this.studentId = studentId;
}
public Add_ress getAddRess() {
return this.addRess;
}
public void setAddRess(Add_ress addRess) {
this.addRess = addRess;
}
public String getStudentName() {
return this.studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
}
package model;
// default package
import java.util.HashSet;
import java.util.Set;
/**
* Add_ress generated by MyEclipse - Hibernate Tools
*/
public class Add_ress implements java.io.Serializable {
// Fields
private Long addressId;
private String addressStreet;
private String addressCity;
private String addressState;
private Long addressPin;
private Set students = new HashSet(0);
// Constructors
/** default constructor */
public Add_ress() {
}
/** full constructor */
public Add_ress(String addressStreet, String addressCity, String addressState, Long addressPin, Set students) {
this.addressStreet = addressStreet;
this.addressCity = addressCity;
this.addressState = addressState;
this.addressPin = addressPin;
this.students = students;
}
// Property accessors
public Long getAddressId() {
return this.addressId;
}
public void setAddressId(Long addressId) {
this.addressId = addressId;
}
public String getAddressStreet() {
return this.addressStreet;
}
public void setAddressStreet(String addressStreet) {
this.addressStreet = addressStreet;
}
public String getAddressCity() {
return this.addressCity;
}
public void setAddressCity(String addressCity) {
this.addressCity = addressCity;
}
public String getAddressState() {
return this.addressState;
}
public void setAddressState(String addressState) {
this.addressState = addressState;
}
public Long getAddressPin() {
return this.addressPin;
}
public void setAddressPin(Long addressPin) {
this.addressPin = addressPin;
}
public Set getStudents() {
return this.students;
}
public void setStudents(Set students) {
this.students = students;
}
}
****************************************************************************************
Mapping Files
****************************************************************************************
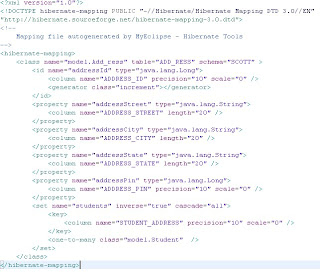
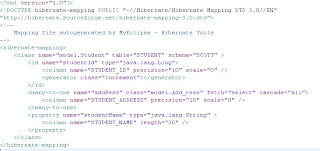
****************************************************************************************
Actual DAO Class
****************************************************************************************
package test;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Set;
import model.Add_ress;
import model.Student;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Session;
import org.hibernate.Transaction;
import factory.HibernateSessionFactory;
public class TestManyToOne {
/**
* @param args
*/
public static void main(String[] args) {
//Saving Address and Student Objects
Add_ress add_ress = new Add_ress();
add_ress.setAddressCity("Yanam");
add_ress.setAddressState("Pondicherry");
add_ress.setAddressStreet("1st Cross");
add_ress.setAddressPin(533464l);
Student student = new Student();
student.setStudentName("Swetha");
Student student1 = new Student();
student1.setStudentName("Nagendra");
student.setAddRess(add_ress);
student1.setAddRess(add_ress);
Set
studentSet.add(student);
studentSet.add(student1);
add_ress.setStudents(studentSet);
// new TestManyToOne().saveStudent(Add_ress.class, add_ress);
//Saving Address and Student Objects
/********************************************************************/
//Getting Address and Student Objects.
/*Add_ress add_ress1 = new Add_ress();
add_ress1.setAddressId(4l);
add_ress1 = (Add_ress)new TestManyToOne().getAddress(Add_ress.class, add_ress1.getAddressId());
System.out.println("City : " + add_ress1.getAddressCity());
System.out.println("State : " + add_ress1.getAddressState());
System.out.println("Street: " + add_ress1.getAddressStreet());
System.out.println("PIN : " + add_ress1.getAddressPin());
studentSet = add_ress1.getStudents();
for (Student student2 : studentSet) {
System.out.println("--------------------------------------------");
System.out.println("Student ID : " + student2.getStudentId());
System.out.println("Student Name: " + student2.getStudentName());
}*/
//Getting Address and Student Objects.
/********************************************************************/
Add_ress add_ress2 = new Add_ress();
Set
Student std1 = new Student();
std1.setStudentId(5l);
Student std2 = new Student();
std2.setStudentId(6l);
stdSet.add(std1);
stdSet.add(std2);
add_ress2.setAddressId(4l);
add_ress2.setStudents(stdSet);
new TestManyToOne().deleteAddress(add_ress2);
}
public void saveStudent(Class clazz, Object object) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Serializable id = session.save(object);
session.close();
tx.commit();
}
public Object getAddress(Class clazz, long id) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Object returnObj = session.get(clazz, id, LockMode.UPGRADE);
return returnObj;
}
public void deleteAddress(Add_ress add_ress) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
session.delete(add_ress);
//session.close();
tx.commit();
}
}
****************************************************************************************
Hibernate - One to Many Example
Tables
****************************************************************************************
create table "SCOTT"."EMPLOYEE"(
"ID" NUMBER(10) not null,
"NAME" VARCHAR2(50),
"DEPT" VARCHAR2(20),
"SAL" NUMBER(10),
constraint "PK_EMPLOYEE" primary key ("ID")
);
create unique index "PK_EMPLOYEE" on "SCOTT"."EMPLOYEE"("ID");
create table "SCOTT"."ADDRESS"(
"ID" NUMBER(10) not null,
"EMP_ID" NUMBER(10),
"STREET" VARCHAR2(30),
"CITY" VARCHAR2(30),
"STATE" VARCHAR2(30),
"PIN" NUMBER(8),
constraint "PK_ADDRESS" primary key ("ID")
);
alter table "SCOTT"."ADDRESS"
add constraint "EMP_ID_FK"
foreign key ("EMP_ID")
references "SCOTT"."EMPLOYEE"("ID");
create unique index "PK_ADDRESS" on "SCOTT"."ADDRESS"("ID");
****************************************************************************************
Hibernate Session Factory Class
****************************************************************************************
package factory;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal threadLocal = new ThreadLocal();
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the
*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
****************************************************************************************
HibernateCFG.xls file
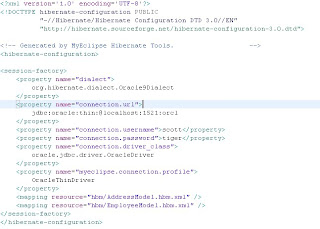
****************************************************************************************
Pojo Classes
****************************************************************************************
package model;
// default package
/**
* AddressModel generated by MyEclipse - Hibernate Tools
*/
public class AddressModel implements java.io.Serializable {
// Fields
private Long id;
private EmployeeModel employee;
private String street;
private String city;
private String state;
private Long pin;
// Constructors
/** default constructor */
public AddressModel() {
}
/** full constructor */
public AddressModel(EmployeeModel employee, String street, String city, String state, Long pin) {
this.employee = employee;
this.street = street;
this.city = city;
this.state = state;
this.pin = pin;
}
// Property accessors
public Long getId() {
return this.id;
}
public void setId(Long id) {
this.id = id;
}
public EmployeeModel getEmployee() {
return this.employee;
}
public void setEmployee(EmployeeModel employee) {
this.employee = employee;
}
public String getStreet() {
return this.street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return this.city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return this.state;
}
public void setState(String state) {
this.state = state;
}
public Long getPin() {
return this.pin;
}
public void setPin(Long pin) {
this.pin = pin;
}
}
package model;
// default package
import java.util.HashSet;
import java.util.Set;
/**
* EmployeeModel generated by MyEclipse - Hibernate Tools
*/
public class EmployeeModel implements java.io.Serializable {
// Fields
private Long id;
private String name;
private String dept;
private Long sal;
private Set addresses = new HashSet(0);
// Constructors
/** default constructor */
public EmployeeModel() {
}
/** full constructor */
public EmployeeModel(String name, String dept, Long sal, Set addresses) {
this.name = name;
this.dept = dept;
this.sal = sal;
this.addresses = addresses;
}
// Property accessors
public Long getId() {
return this.id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public String getDept() {
return this.dept;
}
public void setDept(String dept) {
this.dept = dept;
}
public Long getSal() {
return this.sal;
}
public void setSal(Long sal) {
this.sal = sal;
}
public Set getAddresses() {
return this.addresses;
}
public void setAddresses(Set addresses) {
this.addresses = addresses;
}
}
****************************************************************************************
Mapping files
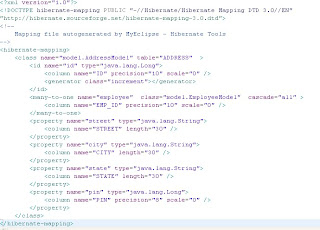
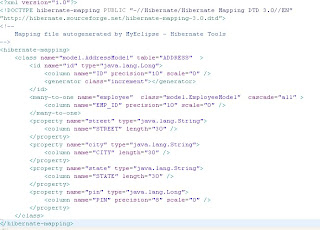
****************************************************************************************
Actual DAO Class
package test;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Set;
import model.AddressModel;
import model.EmployeeModel;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Session;
import org.hibernate.Transaction;
import factory.HibernateSessionFactory;
public class EMPDAO {
/**
* @param args
*/
public static void main(String[] args) {
EmployeeModel employeeModel = new EmployeeModel();
employeeModel.setName("Gangadhara Rao D");
employeeModel.setSal(1000L);
employeeModel.setDept("Java");
Set set = new HashSet();
AddressModel addressModel = new AddressModel();
addressModel.setStreet("3rd Cross");
addressModel.setCity("Bangalore");
addressModel.setState("Karnataka");
addressModel.setPin(560068L);
addressModel.setEmployee(employeeModel);
set.add(addressModel);
employeeModel.setAddresses(set);
employeeModel = (EmployeeModel)new EMPDAO().saveEmployee(EmployeeModel.class, employeeModel);
System.out.println(employeeModel.getId());
}
public Object saveEmployee(Class clazz, Object object) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Serializable id = session.save(object);
tx.commit();
/*session.flush();
session.refresh(object);*/
Object returnObj = session.get(clazz, id, LockMode.UPGRADE);
return returnObj;
}
}
****************************************************************************************
****************************************************************************************
create table "SCOTT"."EMPLOYEE"(
"ID" NUMBER(10) not null,
"NAME" VARCHAR2(50),
"DEPT" VARCHAR2(20),
"SAL" NUMBER(10),
constraint "PK_EMPLOYEE" primary key ("ID")
);
create unique index "PK_EMPLOYEE" on "SCOTT"."EMPLOYEE"("ID");
create table "SCOTT"."ADDRESS"(
"ID" NUMBER(10) not null,
"EMP_ID" NUMBER(10),
"STREET" VARCHAR2(30),
"CITY" VARCHAR2(30),
"STATE" VARCHAR2(30),
"PIN" NUMBER(8),
constraint "PK_ADDRESS" primary key ("ID")
);
alter table "SCOTT"."ADDRESS"
add constraint "EMP_ID_FK"
foreign key ("EMP_ID")
references "SCOTT"."EMPLOYEE"("ID");
create unique index "PK_ADDRESS" on "SCOTT"."ADDRESS"("ID");
****************************************************************************************
Hibernate Session Factory Class
****************************************************************************************
package factory;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the
SessionFactory
if needed.*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
****************************************************************************************
HibernateCFG.xls file
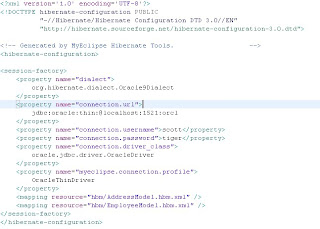
****************************************************************************************
Pojo Classes
****************************************************************************************
package model;
// default package
/**
* AddressModel generated by MyEclipse - Hibernate Tools
*/
public class AddressModel implements java.io.Serializable {
// Fields
private Long id;
private EmployeeModel employee;
private String street;
private String city;
private String state;
private Long pin;
// Constructors
/** default constructor */
public AddressModel() {
}
/** full constructor */
public AddressModel(EmployeeModel employee, String street, String city, String state, Long pin) {
this.employee = employee;
this.street = street;
this.city = city;
this.state = state;
this.pin = pin;
}
// Property accessors
public Long getId() {
return this.id;
}
public void setId(Long id) {
this.id = id;
}
public EmployeeModel getEmployee() {
return this.employee;
}
public void setEmployee(EmployeeModel employee) {
this.employee = employee;
}
public String getStreet() {
return this.street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return this.city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return this.state;
}
public void setState(String state) {
this.state = state;
}
public Long getPin() {
return this.pin;
}
public void setPin(Long pin) {
this.pin = pin;
}
}
package model;
// default package
import java.util.HashSet;
import java.util.Set;
/**
* EmployeeModel generated by MyEclipse - Hibernate Tools
*/
public class EmployeeModel implements java.io.Serializable {
// Fields
private Long id;
private String name;
private String dept;
private Long sal;
private Set addresses = new HashSet(0);
// Constructors
/** default constructor */
public EmployeeModel() {
}
/** full constructor */
public EmployeeModel(String name, String dept, Long sal, Set addresses) {
this.name = name;
this.dept = dept;
this.sal = sal;
this.addresses = addresses;
}
// Property accessors
public Long getId() {
return this.id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public String getDept() {
return this.dept;
}
public void setDept(String dept) {
this.dept = dept;
}
public Long getSal() {
return this.sal;
}
public void setSal(Long sal) {
this.sal = sal;
}
public Set getAddresses() {
return this.addresses;
}
public void setAddresses(Set addresses) {
this.addresses = addresses;
}
}
****************************************************************************************
Mapping files
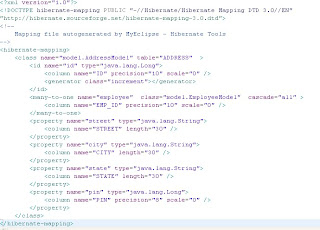
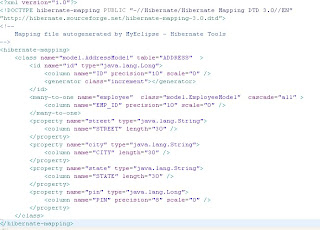
****************************************************************************************
Actual DAO Class
package test;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Set;
import model.AddressModel;
import model.EmployeeModel;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Session;
import org.hibernate.Transaction;
import factory.HibernateSessionFactory;
public class EMPDAO {
/**
* @param args
*/
public static void main(String[] args) {
EmployeeModel employeeModel = new EmployeeModel();
employeeModel.setName("Gangadhara Rao D");
employeeModel.setSal(1000L);
employeeModel.setDept("Java");
Set
AddressModel addressModel = new AddressModel();
addressModel.setStreet("3rd Cross");
addressModel.setCity("Bangalore");
addressModel.setState("Karnataka");
addressModel.setPin(560068L);
addressModel.setEmployee(employeeModel);
set.add(addressModel);
employeeModel.setAddresses(set);
employeeModel = (EmployeeModel)new EMPDAO().saveEmployee(EmployeeModel.class, employeeModel);
System.out.println(employeeModel.getId());
}
public Object saveEmployee(Class clazz, Object object) throws HibernateException {
Session session = HibernateSessionFactory.getSession();
Transaction tx = session.beginTransaction();
Serializable id = session.save(object);
tx.commit();
/*session.flush();
session.refresh(object);*/
Object returnObj = session.get(clazz, id, LockMode.UPGRADE);
return returnObj;
}
}
****************************************************************************************
Monday, February 15, 2010
EJB3 Session Beans
**************************************************************************************
Server Side Code
**************************************************************************************
package test;
import javax.ejb.Remote;
@Remote
public interface TestEJBBean {
public void executeBusinessLogic();
}
**************************************************************************************
package test;
import javax.ejb.Remote;
import javax.ejb.Stateless;
import org.jboss.annotation.ejb.LocalBinding;
import org.jboss.annotation.ejb.RemoteBinding;
@Stateless (name="TestEJBBeanBean")
@Remote(TestEJBBean.class)
@RemoteBinding(jndiBinding = "/ejb3/TestEJBBeanBean")
public class TestEJBBeanBean implements TestEJBBean{
public void executeBusinessLogic() {
System.out.println("Executing business logic.");
}
}
**************************************************************************************
Client Side Code (Create this as another project)
**************************************************************************************
package test;
public interface TestEJBBean {
public void executeBusinessLogic();
}
**************************************************************************************
package client;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Properties;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import test.TestEJBBean;
public class EJBTestClient {
static Properties props = new Properties();
/**
* @param args
*/
public static void main(String[] args) {
try {
props.load(new FileInputStream("jndi.properties"));
InitialContext ic = new InitialContext(props);
TestEJBBean testEJBBean = (TestEJBBean)ic.lookup("/ejb3/TestEJBBeanBean");
testEJBBean.executeBusinessLogic();
} catch(FileNotFoundException fnfe) {
fnfe.printStackTrace();
} catch(IOException ioe) {
ioe.printStackTrace();
} catch(NamingException ne) {
ne.printStackTrace();
}
}
}
**************************************************************************************
EJBJNDI.properties file (for JBoss)
**************************************************************************************
java.naming.factory.initial=org.jnp.interfaces.NamingContextFactory
java.naming.factory.url.pkgs=org.jboss.naming:org.jnp.interfaces
java.naming.provider.url=localhost:1099
TestEJBBeanBean=/ejb3/TestEJBBeanBean
**************************************************************************************
Server Side Code
**************************************************************************************
package test;
import javax.ejb.Remote;
@Remote
public interface TestEJBBean {
public void executeBusinessLogic();
}
**************************************************************************************
package test;
import javax.ejb.Remote;
import javax.ejb.Stateless;
import org.jboss.annotation.ejb.LocalBinding;
import org.jboss.annotation.ejb.RemoteBinding;
@Stateless (name="TestEJBBeanBean")
@Remote(TestEJBBean.class)
@RemoteBinding(jndiBinding = "/ejb3/TestEJBBeanBean")
public class TestEJBBeanBean implements TestEJBBean{
public void executeBusinessLogic() {
System.out.println("Executing business logic.");
}
}
**************************************************************************************
Client Side Code (Create this as another project)
**************************************************************************************
package test;
public interface TestEJBBean {
public void executeBusinessLogic();
}
**************************************************************************************
package client;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Properties;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import test.TestEJBBean;
public class EJBTestClient {
static Properties props = new Properties();
/**
* @param args
*/
public static void main(String[] args) {
try {
props.load(new FileInputStream("jndi.properties"));
InitialContext ic = new InitialContext(props);
TestEJBBean testEJBBean = (TestEJBBean)ic.lookup("/ejb3/TestEJBBeanBean");
testEJBBean.executeBusinessLogic();
} catch(FileNotFoundException fnfe) {
fnfe.printStackTrace();
} catch(IOException ioe) {
ioe.printStackTrace();
} catch(NamingException ne) {
ne.printStackTrace();
}
}
}
**************************************************************************************
EJBJNDI.properties file (for JBoss)
**************************************************************************************
java.naming.factory.initial=org.jnp.interfaces.NamingContextFactory
java.naming.factory.url.pkgs=org.jboss.naming:org.jnp.interfaces
java.naming.provider.url=localhost:1099
TestEJBBeanBean=/ejb3/TestEJBBeanBean
**************************************************************************************
Web Service Complete Program
--------------------------------------------------------------------------------------
Server Side Code
--------------------------------------------------------------------------------------
package home.object;
public class Address {
private String street;
private String city;
private Integer pin;
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public Integer getPin() {
return pin;
}
public void setPin(Integer pin) {
this.pin = pin;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
}
--------------------------------------------------------------------------------------
package home.object;
public class Employee {
private String name;
private Integer empNo;
private Address address;
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public Integer getEmpNo() {
return empNo;
}
public void setEmpNo(Integer empNo) {
this.empNo = empNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
--------------------------------------------------------------------------------------
package home.webservice;
import home.object.Employee;
/**
* @author Soumya Sree
*
*/
public interface EmployeeInterface {
public void displayEmp(Employee emp);
}
-------------------------------------------------------------------------------------
package home.webservice;
import home.object.Address;
import home.object.Employee;
import javax.ejb.Stateless;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import org.jboss.wsf.spi.annotation.WebContext;
@WebService(name = "EmployeeWebService", targetNamespace = "http://object.home/")
@SOAPBinding(parameterStyle = SOAPBinding.ParameterStyle.BARE)
@WebContext(contextRoot = "/empoperations", urlPattern = "/displayEmp")
@Stateless()
public class EmployeeWebService implements EmployeeInterface{
@WebMethod(operationName = "displayEmp", action = "displayEmp")
public void displayEmp(@WebParam(name = "Employee", targetNamespace = "http://object.home/", partName = "employee") Employee emp) {
System.out.println("Employee Name : " + emp.getName());
System.out.println("Employee Number : " + emp.getEmpNo());
Address address = emp.getAddress();
System.out.println("Street : " + address.getStreet());
System.out.println("City : " + address.getCity());
System.out.println("PIN : " + address.getPin());
}
}
-------------------------------------------------------------------------------------
Create the Jar with the help of above files and deploy it into application server, the server automatically creates the WSDL (Web Service Description Language) file, with the help of wsdl and wsdl2java.jar we can write the client side code.
Generated wsdl is looks like the below content.
--------------------------------------------------------------------------------------
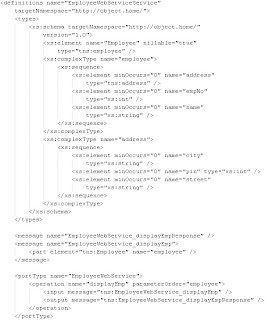
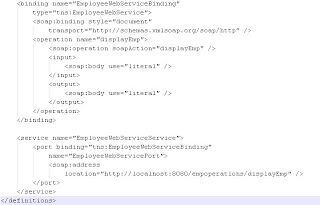
--------------------------------------------------------------------------------------
Client Side Code (Generated with the help of wsdl2java.jar file)
--------------------------------------------------------------------------------------
/**
* Address.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class Address implements java.io.Serializable {
private java.lang.String city;
private java.lang.Integer pin;
private java.lang.String street;
public Address() {
}
public java.lang.String getCity() {
return city;
}
public void setCity(java.lang.String city) {
this.city = city;
}
public java.lang.Integer getPin() {
return pin;
}
public void setPin(java.lang.Integer pin) {
this.pin = pin;
}
public java.lang.String getStreet() {
return street;
}
public void setStreet(java.lang.String street) {
this.street = street;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof Address)) return false;
Address other = (Address) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
((this.city==null && other.getCity()==null) ||
(this.city!=null &&
this.city.equals(other.getCity()))) &&
((this.pin==null && other.getPin()==null) ||
(this.pin!=null &&
this.pin.equals(other.getPin()))) &&
((this.street==null && other.getStreet()==null) ||
(this.street!=null &&
this.street.equals(other.getStreet())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
if (getCity() != null) {
_hashCode += getCity().hashCode();
}
if (getPin() != null) {
_hashCode += getPin().hashCode();
}
if (getStreet() != null) {
_hashCode += getStreet().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(Address.class);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://object.home/", "address"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("city");
elemField.setXmlName(new javax.xml.namespace.QName("", "city"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("pin");
elemField.setXmlName(new javax.xml.namespace.QName("", "pin"));
elemField.setXmlType(new javax.xml.namespace.QName("http://schemas.xmlsoap.org/soap/encoding/", "int"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("street");
elemField.setXmlName(new javax.xml.namespace.QName("", "street"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
--------------------------------------------------------------------------------------
/**
* Employee.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class Employee implements java.io.Serializable {
private home.object.Address address;
private java.lang.Integer empNo;
private java.lang.String name;
public Employee() {
}
public home.object.Address getAddress() {
return address;
}
public void setAddress(home.object.Address address) {
this.address = address;
}
public java.lang.Integer getEmpNo() {
return empNo;
}
public void setEmpNo(java.lang.Integer empNo) {
this.empNo = empNo;
}
public java.lang.String getName() {
return name;
}
public void setName(java.lang.String name) {
this.name = name;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof Employee)) return false;
Employee other = (Employee) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
((this.address==null && other.getAddress()==null) ||
(this.address!=null &&
this.address.equals(other.getAddress()))) &&
((this.empNo==null && other.getEmpNo()==null) ||
(this.empNo!=null &&
this.empNo.equals(other.getEmpNo()))) &&
((this.name==null && other.getName()==null) ||
(this.name!=null &&
this.name.equals(other.getName())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
if (getAddress() != null) {
_hashCode += getAddress().hashCode();
}
if (getEmpNo() != null) {
_hashCode += getEmpNo().hashCode();
}
if (getName() != null) {
_hashCode += getName().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(Employee.class);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://object.home/", "employee"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("address");
elemField.setXmlName(new javax.xml.namespace.QName("", "address"));
elemField.setXmlType(new javax.xml.namespace.QName("http://object.home/", "address"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("empNo");
elemField.setXmlName(new javax.xml.namespace.QName("", "empNo"));
elemField.setXmlType(new javax.xml.namespace.QName("http://schemas.xmlsoap.org/soap/encoding/", "int"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("name");
elemField.setXmlName(new javax.xml.namespace.QName("", "name"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebService.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public interface EmployeeWebService extends java.rmi.Remote {
public void displayEmp(home.object.Employee employee) throws java.rmi.RemoteException;
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebServiceBindingStub.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class EmployeeWebServiceBindingStub extends org.apache.axis.client.Stub implements home.object.EmployeeWebService {
private java.util.Vector cachedSerClasses = new java.util.Vector();
private java.util.Vector cachedSerQNames = new java.util.Vector();
private java.util.Vector cachedSerFactories = new java.util.Vector();
private java.util.Vector cachedDeserFactories = new java.util.Vector();
static org.apache.axis.description.OperationDesc [] _operations;
static {
_operations = new org.apache.axis.description.OperationDesc[1];
org.apache.axis.description.OperationDesc oper;
oper = new org.apache.axis.description.OperationDesc();
oper.setName("displayEmp");
oper.addParameter(new javax.xml.namespace.QName("http://object.home/", "Employee"), new javax.xml.namespace.QName("http://object.home/", "employee"), home.object.Employee.class, org.apache.axis.description.ParameterDesc.IN, false, false);
oper.setReturnType(org.apache.axis.encoding.XMLType.AXIS_VOID);
oper.setStyle(org.apache.axis.constants.Style.DOCUMENT);
oper.setUse(org.apache.axis.constants.Use.LITERAL);
_operations[0] = oper;
}
public EmployeeWebServiceBindingStub() throws org.apache.axis.AxisFault {
this(null);
}
public EmployeeWebServiceBindingStub(java.net.URL endpointURL, javax.xml.rpc.Service service) throws org.apache.axis.AxisFault {
this(service);
super.cachedEndpoint = endpointURL;
}
public EmployeeWebServiceBindingStub(javax.xml.rpc.Service service) throws org.apache.axis.AxisFault {
if (service == null) {
super.service = new org.apache.axis.client.Service();
} else {
super.service = service;
}
java.lang.Class cls;
javax.xml.namespace.QName qName;
java.lang.Class beansf = org.apache.axis.encoding.ser.BeanSerializerFactory.class;
java.lang.Class beandf = org.apache.axis.encoding.ser.BeanDeserializerFactory.class;
java.lang.Class enumsf = org.apache.axis.encoding.ser.EnumSerializerFactory.class;
java.lang.Class enumdf = org.apache.axis.encoding.ser.EnumDeserializerFactory.class;
java.lang.Class arraysf = org.apache.axis.encoding.ser.ArraySerializerFactory.class;
java.lang.Class arraydf = org.apache.axis.encoding.ser.ArrayDeserializerFactory.class;
java.lang.Class simplesf = org.apache.axis.encoding.ser.SimpleSerializerFactory.class;
java.lang.Class simpledf = org.apache.axis.encoding.ser.SimpleDeserializerFactory.class;
qName = new javax.xml.namespace.QName("http://object.home/", "address");
cachedSerQNames.add(qName);
cls = home.object.Address.class;
cachedSerClasses.add(cls);
cachedSerFactories.add(beansf);
cachedDeserFactories.add(beandf);
qName = new javax.xml.namespace.QName("http://object.home/", "employee");
cachedSerQNames.add(qName);
cls = home.object.Employee.class;
cachedSerClasses.add(cls);
cachedSerFactories.add(beansf);
cachedDeserFactories.add(beandf);
}
private org.apache.axis.client.Call createCall() throws java.rmi.RemoteException {
try {
org.apache.axis.client.Call _call =
(org.apache.axis.client.Call) super.service.createCall();
if (super.maintainSessionSet) {
_call.setMaintainSession(super.maintainSession);
}
if (super.cachedUsername != null) {
_call.setUsername(super.cachedUsername);
}
if (super.cachedPassword != null) {
_call.setPassword(super.cachedPassword);
}
if (super.cachedEndpoint != null) {
_call.setTargetEndpointAddress(super.cachedEndpoint);
}
if (super.cachedTimeout != null) {
_call.setTimeout(super.cachedTimeout);
}
if (super.cachedPortName != null) {
_call.setPortName(super.cachedPortName);
}
java.util.Enumeration keys = super.cachedProperties.keys();
while (keys.hasMoreElements()) {
java.lang.String key = (java.lang.String) keys.nextElement();
_call.setProperty(key, super.cachedProperties.get(key));
}
// All the type mapping information is registered
// when the first call is made.
// The type mapping information is actually registered in
// the TypeMappingRegistry of the service, which
// is the reason why registration is only needed for the first call.
synchronized (this) {
if (firstCall()) {
// must set encoding style before registering serializers
_call.setEncodingStyle(null);
for (int i = 0; i < cachedSerFactories.size(); ++i) {
java.lang.Class cls = (java.lang.Class) cachedSerClasses.get(i);
javax.xml.namespace.QName qName =
(javax.xml.namespace.QName) cachedSerQNames.get(i);
java.lang.Class sf = (java.lang.Class)
cachedSerFactories.get(i);
java.lang.Class df = (java.lang.Class)
cachedDeserFactories.get(i);
_call.registerTypeMapping(cls, qName, sf, df, false);
}
}
}
return _call;
}
catch (java.lang.Throwable t) {
throw new org.apache.axis.AxisFault("Failure trying to get the Call object", t);
}
}
public void displayEmp(home.object.Employee employee) throws java.rmi.RemoteException {
if (super.cachedEndpoint == null) {
throw new org.apache.axis.NoEndPointException();
}
org.apache.axis.client.Call _call = createCall();
_call.setOperation(_operations[0]);
_call.setUseSOAPAction(true);
_call.setSOAPActionURI("displayEmp");
_call.setEncodingStyle(null);
_call.setProperty(org.apache.axis.client.Call.SEND_TYPE_ATTR, Boolean.FALSE);
_call.setProperty(org.apache.axis.AxisEngine.PROP_DOMULTIREFS, Boolean.FALSE);
_call.setSOAPVersion(org.apache.axis.soap.SOAPConstants.SOAP11_CONSTANTS);
_call.setOperationName(new javax.xml.namespace.QName("", "displayEmp"));
setRequestHeaders(_call);
setAttachments(_call);
java.lang.Object _resp = _call.invoke(new java.lang.Object[] {employee});
if (_resp instanceof java.rmi.RemoteException) {
throw (java.rmi.RemoteException)_resp;
}
extractAttachments(_call);
}
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebServiceService.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public interface EmployeeWebServiceService extends javax.xml.rpc.Service {
public java.lang.String getEmployeeWebServicePortAddress();
public home.object.EmployeeWebService getEmployeeWebServicePort() throws javax.xml.rpc.ServiceException;
public home.object.EmployeeWebService getEmployeeWebServicePort(java.net.URL portAddress) throws javax.xml.rpc.ServiceException;
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebServiceServiceLocator.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class EmployeeWebServiceServiceLocator extends org.apache.axis.client.Service implements home.object.EmployeeWebServiceService {
// Use to get a proxy class for EmployeeWebServicePort
private final java.lang.String EmployeeWebServicePort_address = "http://127.0.0.1:8080/empoperations/displayEmp";
public java.lang.String getEmployeeWebServicePortAddress() {
return EmployeeWebServicePort_address;
}
// The WSDD service name defaults to the port name.
private java.lang.String EmployeeWebServicePortWSDDServiceName = "EmployeeWebServicePort";
public java.lang.String getEmployeeWebServicePortWSDDServiceName() {
return EmployeeWebServicePortWSDDServiceName;
}
public void setEmployeeWebServicePortWSDDServiceName(java.lang.String name) {
EmployeeWebServicePortWSDDServiceName = name;
}
public home.object.EmployeeWebService getEmployeeWebServicePort() throws javax.xml.rpc.ServiceException {
java.net.URL endpoint;
try {
endpoint = new java.net.URL(EmployeeWebServicePort_address);
}
catch (java.net.MalformedURLException e) {
throw new javax.xml.rpc.ServiceException(e);
}
return getEmployeeWebServicePort(endpoint);
}
public home.object.EmployeeWebService getEmployeeWebServicePort(java.net.URL portAddress) throws javax.xml.rpc.ServiceException {
try {
home.object.EmployeeWebServiceBindingStub _stub = new home.object.EmployeeWebServiceBindingStub(portAddress, this);
_stub.setPortName(getEmployeeWebServicePortWSDDServiceName());
return _stub;
}
catch (org.apache.axis.AxisFault e) {
return null;
}
}
/**
* For the given interface, get the stub implementation.
* If this service has no port for the given interface,
* then ServiceException is thrown.
*/
public java.rmi.Remote getPort(Class serviceEndpointInterface) throws javax.xml.rpc.ServiceException {
try {
if (home.object.EmployeeWebService.class.isAssignableFrom(serviceEndpointInterface)) {
home.object.EmployeeWebServiceBindingStub _stub = new home.object.EmployeeWebServiceBindingStub(new java.net.URL(EmployeeWebServicePort_address), this);
_stub.setPortName(getEmployeeWebServicePortWSDDServiceName());
return _stub;
}
}
catch (java.lang.Throwable t) {
throw new javax.xml.rpc.ServiceException(t);
}
throw new javax.xml.rpc.ServiceException("There is no stub implementation for the interface: " + (serviceEndpointInterface == null ? "null" : serviceEndpointInterface.getName()));
}
/**
* For the given interface, get the stub implementation.
* If this service has no port for the given interface,
* then ServiceException is thrown.
*/
public java.rmi.Remote getPort(javax.xml.namespace.QName portName, Class serviceEndpointInterface) throws javax.xml.rpc.ServiceException {
if (portName == null) {
return getPort(serviceEndpointInterface);
}
String inputPortName = portName.getLocalPart();
if ("EmployeeWebServicePort".equals(inputPortName)) {
return getEmployeeWebServicePort();
}
else {
java.rmi.Remote _stub = getPort(serviceEndpointInterface);
((org.apache.axis.client.Stub) _stub).setPortName(portName);
return _stub;
}
}
public javax.xml.namespace.QName getServiceName() {
return new javax.xml.namespace.QName("http://object.home/", "EmployeeWebServiceService");
}
private java.util.HashSet ports = null;
public java.util.Iterator getPorts() {
if (ports == null) {
ports = new java.util.HashSet();
ports.add(new javax.xml.namespace.QName("EmployeeWebServicePort"));
}
return ports.iterator();
}
}
--------------------------------------------------------------------------------------
Actual Client Program this is the one which initiates locator and access the server
--------------------------------------------------------------------------------------
package home.object;
import java.net.MalformedURLException;
import java.rmi.RemoteException;
import org.apache.axis.AxisFault;
public class Client {
/**
* @param args
*/
public static void main(String[] args) {
Employee employee = new Employee();
employee.setName("Gangadhara Rao D");
employee.setEmpNo(10662);
Address address = new Address();
address.setStreet("3rd Cross");
address.setCity("Bangalore");
address.setPin(111111);
employee.setAddress(address);
try {
java.net.URL url = new java.net.URL("http://127.0.0.1:8080/empoperations/displayEmp");
EmployeeWebServiceBindingStub employeeWebServiceBindingStub = new EmployeeWebServiceBindingStub(url, new org.apache.axis.client.Service());
employeeWebServiceBindingStub.displayEmp(employee);
} catch(MalformedURLException mfurle) {
mfurle.printStackTrace();
} catch(AxisFault af) {
af.printStackTrace();
} catch(RemoteException re) {
re.printStackTrace();
}
}
}
--------------------------------------------------------------------------------------
Server Side Code
--------------------------------------------------------------------------------------
package home.object;
public class Address {
private String street;
private String city;
private Integer pin;
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public Integer getPin() {
return pin;
}
public void setPin(Integer pin) {
this.pin = pin;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
}
--------------------------------------------------------------------------------------
package home.object;
public class Employee {
private String name;
private Integer empNo;
private Address address;
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public Integer getEmpNo() {
return empNo;
}
public void setEmpNo(Integer empNo) {
this.empNo = empNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
--------------------------------------------------------------------------------------
package home.webservice;
import home.object.Employee;
/**
* @author Soumya Sree
*
*/
public interface EmployeeInterface {
public void displayEmp(Employee emp);
}
-------------------------------------------------------------------------------------
package home.webservice;
import home.object.Address;
import home.object.Employee;
import javax.ejb.Stateless;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import org.jboss.wsf.spi.annotation.WebContext;
@WebService(name = "EmployeeWebService", targetNamespace = "http://object.home/")
@SOAPBinding(parameterStyle = SOAPBinding.ParameterStyle.BARE)
@WebContext(contextRoot = "/empoperations", urlPattern = "/displayEmp")
@Stateless()
public class EmployeeWebService implements EmployeeInterface{
@WebMethod(operationName = "displayEmp", action = "displayEmp")
public void displayEmp(@WebParam(name = "Employee", targetNamespace = "http://object.home/", partName = "employee") Employee emp) {
System.out.println("Employee Name : " + emp.getName());
System.out.println("Employee Number : " + emp.getEmpNo());
Address address = emp.getAddress();
System.out.println("Street : " + address.getStreet());
System.out.println("City : " + address.getCity());
System.out.println("PIN : " + address.getPin());
}
}
-------------------------------------------------------------------------------------
Create the Jar with the help of above files and deploy it into application server, the server automatically creates the WSDL (Web Service Description Language) file, with the help of wsdl and wsdl2java.jar we can write the client side code.
Generated wsdl is looks like the below content.
--------------------------------------------------------------------------------------
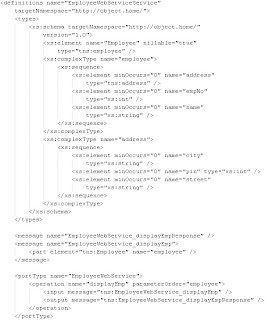
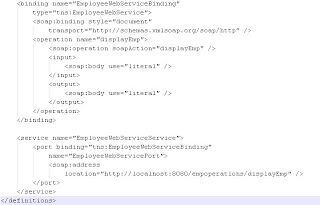
--------------------------------------------------------------------------------------
Client Side Code (Generated with the help of wsdl2java.jar file)
--------------------------------------------------------------------------------------
/**
* Address.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class Address implements java.io.Serializable {
private java.lang.String city;
private java.lang.Integer pin;
private java.lang.String street;
public Address() {
}
public java.lang.String getCity() {
return city;
}
public void setCity(java.lang.String city) {
this.city = city;
}
public java.lang.Integer getPin() {
return pin;
}
public void setPin(java.lang.Integer pin) {
this.pin = pin;
}
public java.lang.String getStreet() {
return street;
}
public void setStreet(java.lang.String street) {
this.street = street;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof Address)) return false;
Address other = (Address) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
((this.city==null && other.getCity()==null) ||
(this.city!=null &&
this.city.equals(other.getCity()))) &&
((this.pin==null && other.getPin()==null) ||
(this.pin!=null &&
this.pin.equals(other.getPin()))) &&
((this.street==null && other.getStreet()==null) ||
(this.street!=null &&
this.street.equals(other.getStreet())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
if (getCity() != null) {
_hashCode += getCity().hashCode();
}
if (getPin() != null) {
_hashCode += getPin().hashCode();
}
if (getStreet() != null) {
_hashCode += getStreet().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(Address.class);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://object.home/", "address"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("city");
elemField.setXmlName(new javax.xml.namespace.QName("", "city"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("pin");
elemField.setXmlName(new javax.xml.namespace.QName("", "pin"));
elemField.setXmlType(new javax.xml.namespace.QName("http://schemas.xmlsoap.org/soap/encoding/", "int"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("street");
elemField.setXmlName(new javax.xml.namespace.QName("", "street"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
--------------------------------------------------------------------------------------
/**
* Employee.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class Employee implements java.io.Serializable {
private home.object.Address address;
private java.lang.Integer empNo;
private java.lang.String name;
public Employee() {
}
public home.object.Address getAddress() {
return address;
}
public void setAddress(home.object.Address address) {
this.address = address;
}
public java.lang.Integer getEmpNo() {
return empNo;
}
public void setEmpNo(java.lang.Integer empNo) {
this.empNo = empNo;
}
public java.lang.String getName() {
return name;
}
public void setName(java.lang.String name) {
this.name = name;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof Employee)) return false;
Employee other = (Employee) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
((this.address==null && other.getAddress()==null) ||
(this.address!=null &&
this.address.equals(other.getAddress()))) &&
((this.empNo==null && other.getEmpNo()==null) ||
(this.empNo!=null &&
this.empNo.equals(other.getEmpNo()))) &&
((this.name==null && other.getName()==null) ||
(this.name!=null &&
this.name.equals(other.getName())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
if (getAddress() != null) {
_hashCode += getAddress().hashCode();
}
if (getEmpNo() != null) {
_hashCode += getEmpNo().hashCode();
}
if (getName() != null) {
_hashCode += getName().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(Employee.class);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://object.home/", "employee"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("address");
elemField.setXmlName(new javax.xml.namespace.QName("", "address"));
elemField.setXmlType(new javax.xml.namespace.QName("http://object.home/", "address"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("empNo");
elemField.setXmlName(new javax.xml.namespace.QName("", "empNo"));
elemField.setXmlType(new javax.xml.namespace.QName("http://schemas.xmlsoap.org/soap/encoding/", "int"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("name");
elemField.setXmlName(new javax.xml.namespace.QName("", "name"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebService.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public interface EmployeeWebService extends java.rmi.Remote {
public void displayEmp(home.object.Employee employee) throws java.rmi.RemoteException;
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebServiceBindingStub.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class EmployeeWebServiceBindingStub extends org.apache.axis.client.Stub implements home.object.EmployeeWebService {
private java.util.Vector cachedSerClasses = new java.util.Vector();
private java.util.Vector cachedSerQNames = new java.util.Vector();
private java.util.Vector cachedSerFactories = new java.util.Vector();
private java.util.Vector cachedDeserFactories = new java.util.Vector();
static org.apache.axis.description.OperationDesc [] _operations;
static {
_operations = new org.apache.axis.description.OperationDesc[1];
org.apache.axis.description.OperationDesc oper;
oper = new org.apache.axis.description.OperationDesc();
oper.setName("displayEmp");
oper.addParameter(new javax.xml.namespace.QName("http://object.home/", "Employee"), new javax.xml.namespace.QName("http://object.home/", "employee"), home.object.Employee.class, org.apache.axis.description.ParameterDesc.IN, false, false);
oper.setReturnType(org.apache.axis.encoding.XMLType.AXIS_VOID);
oper.setStyle(org.apache.axis.constants.Style.DOCUMENT);
oper.setUse(org.apache.axis.constants.Use.LITERAL);
_operations[0] = oper;
}
public EmployeeWebServiceBindingStub() throws org.apache.axis.AxisFault {
this(null);
}
public EmployeeWebServiceBindingStub(java.net.URL endpointURL, javax.xml.rpc.Service service) throws org.apache.axis.AxisFault {
this(service);
super.cachedEndpoint = endpointURL;
}
public EmployeeWebServiceBindingStub(javax.xml.rpc.Service service) throws org.apache.axis.AxisFault {
if (service == null) {
super.service = new org.apache.axis.client.Service();
} else {
super.service = service;
}
java.lang.Class cls;
javax.xml.namespace.QName qName;
java.lang.Class beansf = org.apache.axis.encoding.ser.BeanSerializerFactory.class;
java.lang.Class beandf = org.apache.axis.encoding.ser.BeanDeserializerFactory.class;
java.lang.Class enumsf = org.apache.axis.encoding.ser.EnumSerializerFactory.class;
java.lang.Class enumdf = org.apache.axis.encoding.ser.EnumDeserializerFactory.class;
java.lang.Class arraysf = org.apache.axis.encoding.ser.ArraySerializerFactory.class;
java.lang.Class arraydf = org.apache.axis.encoding.ser.ArrayDeserializerFactory.class;
java.lang.Class simplesf = org.apache.axis.encoding.ser.SimpleSerializerFactory.class;
java.lang.Class simpledf = org.apache.axis.encoding.ser.SimpleDeserializerFactory.class;
qName = new javax.xml.namespace.QName("http://object.home/", "address");
cachedSerQNames.add(qName);
cls = home.object.Address.class;
cachedSerClasses.add(cls);
cachedSerFactories.add(beansf);
cachedDeserFactories.add(beandf);
qName = new javax.xml.namespace.QName("http://object.home/", "employee");
cachedSerQNames.add(qName);
cls = home.object.Employee.class;
cachedSerClasses.add(cls);
cachedSerFactories.add(beansf);
cachedDeserFactories.add(beandf);
}
private org.apache.axis.client.Call createCall() throws java.rmi.RemoteException {
try {
org.apache.axis.client.Call _call =
(org.apache.axis.client.Call) super.service.createCall();
if (super.maintainSessionSet) {
_call.setMaintainSession(super.maintainSession);
}
if (super.cachedUsername != null) {
_call.setUsername(super.cachedUsername);
}
if (super.cachedPassword != null) {
_call.setPassword(super.cachedPassword);
}
if (super.cachedEndpoint != null) {
_call.setTargetEndpointAddress(super.cachedEndpoint);
}
if (super.cachedTimeout != null) {
_call.setTimeout(super.cachedTimeout);
}
if (super.cachedPortName != null) {
_call.setPortName(super.cachedPortName);
}
java.util.Enumeration keys = super.cachedProperties.keys();
while (keys.hasMoreElements()) {
java.lang.String key = (java.lang.String) keys.nextElement();
_call.setProperty(key, super.cachedProperties.get(key));
}
// All the type mapping information is registered
// when the first call is made.
// The type mapping information is actually registered in
// the TypeMappingRegistry of the service, which
// is the reason why registration is only needed for the first call.
synchronized (this) {
if (firstCall()) {
// must set encoding style before registering serializers
_call.setEncodingStyle(null);
for (int i = 0; i < cachedSerFactories.size(); ++i) {
java.lang.Class cls = (java.lang.Class) cachedSerClasses.get(i);
javax.xml.namespace.QName qName =
(javax.xml.namespace.QName) cachedSerQNames.get(i);
java.lang.Class sf = (java.lang.Class)
cachedSerFactories.get(i);
java.lang.Class df = (java.lang.Class)
cachedDeserFactories.get(i);
_call.registerTypeMapping(cls, qName, sf, df, false);
}
}
}
return _call;
}
catch (java.lang.Throwable t) {
throw new org.apache.axis.AxisFault("Failure trying to get the Call object", t);
}
}
public void displayEmp(home.object.Employee employee) throws java.rmi.RemoteException {
if (super.cachedEndpoint == null) {
throw new org.apache.axis.NoEndPointException();
}
org.apache.axis.client.Call _call = createCall();
_call.setOperation(_operations[0]);
_call.setUseSOAPAction(true);
_call.setSOAPActionURI("displayEmp");
_call.setEncodingStyle(null);
_call.setProperty(org.apache.axis.client.Call.SEND_TYPE_ATTR, Boolean.FALSE);
_call.setProperty(org.apache.axis.AxisEngine.PROP_DOMULTIREFS, Boolean.FALSE);
_call.setSOAPVersion(org.apache.axis.soap.SOAPConstants.SOAP11_CONSTANTS);
_call.setOperationName(new javax.xml.namespace.QName("", "displayEmp"));
setRequestHeaders(_call);
setAttachments(_call);
java.lang.Object _resp = _call.invoke(new java.lang.Object[] {employee});
if (_resp instanceof java.rmi.RemoteException) {
throw (java.rmi.RemoteException)_resp;
}
extractAttachments(_call);
}
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebServiceService.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public interface EmployeeWebServiceService extends javax.xml.rpc.Service {
public java.lang.String getEmployeeWebServicePortAddress();
public home.object.EmployeeWebService getEmployeeWebServicePort() throws javax.xml.rpc.ServiceException;
public home.object.EmployeeWebService getEmployeeWebServicePort(java.net.URL portAddress) throws javax.xml.rpc.ServiceException;
}
--------------------------------------------------------------------------------------
/**
* EmployeeWebServiceServiceLocator.java
*
* This file was auto-generated from WSDL
* by the Apache Axis WSDL2Java emitter.
*/
package home.object;
public class EmployeeWebServiceServiceLocator extends org.apache.axis.client.Service implements home.object.EmployeeWebServiceService {
// Use to get a proxy class for EmployeeWebServicePort
private final java.lang.String EmployeeWebServicePort_address = "http://127.0.0.1:8080/empoperations/displayEmp";
public java.lang.String getEmployeeWebServicePortAddress() {
return EmployeeWebServicePort_address;
}
// The WSDD service name defaults to the port name.
private java.lang.String EmployeeWebServicePortWSDDServiceName = "EmployeeWebServicePort";
public java.lang.String getEmployeeWebServicePortWSDDServiceName() {
return EmployeeWebServicePortWSDDServiceName;
}
public void setEmployeeWebServicePortWSDDServiceName(java.lang.String name) {
EmployeeWebServicePortWSDDServiceName = name;
}
public home.object.EmployeeWebService getEmployeeWebServicePort() throws javax.xml.rpc.ServiceException {
java.net.URL endpoint;
try {
endpoint = new java.net.URL(EmployeeWebServicePort_address);
}
catch (java.net.MalformedURLException e) {
throw new javax.xml.rpc.ServiceException(e);
}
return getEmployeeWebServicePort(endpoint);
}
public home.object.EmployeeWebService getEmployeeWebServicePort(java.net.URL portAddress) throws javax.xml.rpc.ServiceException {
try {
home.object.EmployeeWebServiceBindingStub _stub = new home.object.EmployeeWebServiceBindingStub(portAddress, this);
_stub.setPortName(getEmployeeWebServicePortWSDDServiceName());
return _stub;
}
catch (org.apache.axis.AxisFault e) {
return null;
}
}
/**
* For the given interface, get the stub implementation.
* If this service has no port for the given interface,
* then ServiceException is thrown.
*/
public java.rmi.Remote getPort(Class serviceEndpointInterface) throws javax.xml.rpc.ServiceException {
try {
if (home.object.EmployeeWebService.class.isAssignableFrom(serviceEndpointInterface)) {
home.object.EmployeeWebServiceBindingStub _stub = new home.object.EmployeeWebServiceBindingStub(new java.net.URL(EmployeeWebServicePort_address), this);
_stub.setPortName(getEmployeeWebServicePortWSDDServiceName());
return _stub;
}
}
catch (java.lang.Throwable t) {
throw new javax.xml.rpc.ServiceException(t);
}
throw new javax.xml.rpc.ServiceException("There is no stub implementation for the interface: " + (serviceEndpointInterface == null ? "null" : serviceEndpointInterface.getName()));
}
/**
* For the given interface, get the stub implementation.
* If this service has no port for the given interface,
* then ServiceException is thrown.
*/
public java.rmi.Remote getPort(javax.xml.namespace.QName portName, Class serviceEndpointInterface) throws javax.xml.rpc.ServiceException {
if (portName == null) {
return getPort(serviceEndpointInterface);
}
String inputPortName = portName.getLocalPart();
if ("EmployeeWebServicePort".equals(inputPortName)) {
return getEmployeeWebServicePort();
}
else {
java.rmi.Remote _stub = getPort(serviceEndpointInterface);
((org.apache.axis.client.Stub) _stub).setPortName(portName);
return _stub;
}
}
public javax.xml.namespace.QName getServiceName() {
return new javax.xml.namespace.QName("http://object.home/", "EmployeeWebServiceService");
}
private java.util.HashSet ports = null;
public java.util.Iterator getPorts() {
if (ports == null) {
ports = new java.util.HashSet();
ports.add(new javax.xml.namespace.QName("EmployeeWebServicePort"));
}
return ports.iterator();
}
}
--------------------------------------------------------------------------------------
Actual Client Program this is the one which initiates locator and access the server
--------------------------------------------------------------------------------------
package home.object;
import java.net.MalformedURLException;
import java.rmi.RemoteException;
import org.apache.axis.AxisFault;
public class Client {
/**
* @param args
*/
public static void main(String[] args) {
Employee employee = new Employee();
employee.setName("Gangadhara Rao D");
employee.setEmpNo(10662);
Address address = new Address();
address.setStreet("3rd Cross");
address.setCity("Bangalore");
address.setPin(111111);
employee.setAddress(address);
try {
java.net.URL url = new java.net.URL("http://127.0.0.1:8080/empoperations/displayEmp");
EmployeeWebServiceBindingStub employeeWebServiceBindingStub = new EmployeeWebServiceBindingStub(url, new org.apache.axis.client.Service());
employeeWebServiceBindingStub.displayEmp(employee);
} catch(MalformedURLException mfurle) {
mfurle.printStackTrace();
} catch(AxisFault af) {
af.printStackTrace();
} catch(RemoteException re) {
re.printStackTrace();
}
}
}
--------------------------------------------------------------------------------------
Monday, June 29, 2009
Message Driven Bean
Message Driven Bean
Message Driven Bean (MDB) is an enterprise bean which runs inside the EJB container and it acts as Listener for the JMS asynchronous message . It does not have Home and Remote interface as Session or Entity bean. It is called by container when container receives JMS asynchronous message. MDB has to implement MessageListener which has a method onMessage(Message msg). When the container calls the MDB it passes the message to onMesage() method and then MDB process that message.
Example Program:
Server Side Program
package org.jboss.tutorial.mdb.bean;
import javax.ejb.ActivationConfigProperty;
import javax.ejb.MessageDriven;
import javax.jms.BytesMessage;
import javax.jms.Message;
import javax.jms.MessageListener;
@MessageDriven(activationConfig = {
@ActivationConfigProperty(propertyName="destinationType", propertyValue="javax.jms.Queue"),
@ActivationConfigProperty(propertyName="destination", propertyValue="queue/tutorial/example")
})
public class ExampleMDB implements MessageListener {
public void onMessage(Message recvMsg) {
System.out.println("----------------");
System.out.println("Received message");
System.out.println("----------------");
System.out.println("Received message object: " + recvMsg);
BytesMessage byteMsg = (BytesMessage) recvMsg;
}
}
Client Side Program
package org.jboss.tutorial.mdb.client;
import java.io.FileInputStream;
import java.util.Properties;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSender;
import javax.jms.QueueSession;
import javax.jms.TextMessage;
import javax.naming.InitialContext;
public class Client {
public static void main(String[] args) throws Exception {
QueueConnection cnn = null;
QueueSender sender = null;
QueueSession session = null;
Properties props = new Properties();
props.load(new FileInputStream("EJBJNDI.prop")); //
InitialContext ic = new InitialContext(props);
//InitialContext ctx = new InitialContext();
Queue queue = (Queue) ic.lookup("queue/tutorial/example");
QueueConnectionFactory factory = (QueueConnectionFactory) ic.lookup("ConnectionFactory");
cnn = factory.createQueueConnection();
session = cnn.createQueueSession(false, QueueSession.AUTO_ACKNOWLEDGE);
TextMessage msg = session.createTextMessage("Hello World");
sender = session.createSender(queue);
sender.send(msg);
System.out.println("Message sent successfully to remote queue.");
}
}
EJBJNDI.prop
java.naming.factory.initial=org.jnp.interfaces.NamingContextFactory
java.naming.factory.url.pkgs=org.jboss.naming:org.jnp.interfaces
java.naming.provider.url=localhost:1099
Subscribe to:
Posts (Atom)